Embedding a Microsoft Office Document in a Java Frame |
|
According to Microsoft Knowledge Base article 242243 and article 311765, Microsoft Office documents (including Word, Excel, PowerPoint, Project, and Visio documents) are ActiveX Document servers, not ActiveX Controls. Visual Basic does not support a native control to host ActiveX Documents. Instead, Visual Basic uses the OLE container control to embed an ActiveX Document. Therefore you cannot directly embed ActiveX Documents in a Java frame either. There are three workarounds:
1. Use the WebBrowser ActiveX Control
The WebBrowser control that comes with Internet Explorer (version 3.0 and higher) does support this form of in-place containment. It is possible to use this control to open an Office document as an ActiveX Document as mentioned in Microsoft Knowledge Base Article - 243058.
Run J-Integra's com2java tool and select C:\WINDOWS\system32\shdocvw.dll as the type library. Check the "Generate extra classes for embedding ActiveX controls in AWT components" option and use shdocvw as the package name. Generate the proxies to a subdirectory named shdocvw.
Note: Due to a method naming conflict between java.awt.Component and shdocvw.WebBrowser, you will receive the errors below when attempting to compile the generated proxies. To correct the conflict, you need to manually rename these methods in WebBrowser.java. For your convenience, an updated version of WebBrowser.java can be downloaded here.
shdocvw\WebBrowser.java:725: getName() in shdocvw.WebBrowser cannot override
getName() in java.awt.Component; overridden method does not throw
com.linar.jintegra.AutomationException
public String getName () throws java.io.IOException, com.linar.jintegra.AutomationException {
^
shdocvw\WebBrowser.java:809: isVisible() in shdocvw.WebBrowser cannot override
isVisible() in java.awt.Component; overridden method does not throw
com.linar.jintegra.AutomationException
public boolean isVisible () throws java.io.IOException, com.linar.jintegra.AutomationException {
^
shdocvw\WebBrowser.java:830: setVisible(boolean) in shdocvw.WebBrowser cannot override
setVisible(boolean) in java.awt.Component; overridden method does not throw
com.linar.jintegra.AutomationException
public void setVisible () throws java.io.IOException, com.linar.jintegra.AutomationException {
^
|
The code below is an example of embedding the WebBrowser Control in a Java Frame.
import shdocvw.*;
import javax.swing.*;
import java.awt.BorderLayout;
import java.awt.event.*;
public class WebBrowserExample extends JFrame {
WebBrowser browser = null;
public WebBrowserExample() {
super("IE Browser");
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
try{
// workaround to cause IE control to release references to
// to editable documents like Word and Excel
browser.navigate(null, null, null, null, null); // go to a blank page
Thread.sleep(1000); // wait for a sec to complete navigation
// it's important to call releaseAll before the application exits
com.linar.jintegra.Cleaner.releaseAll();
System.out.println("application exits");
System.exit(0);
}catch(Exception ex){
ex.printStackTrace();
}
}
});
setBounds(100, 100, 600, 400);
this.getContentPane().setLayout(new BorderLayout());
try {
WebBrowserListener listener = new WebBrowserListener();
browser = new WebBrowser();
browser.addDWebBrowserEvents2Listener(listener);
this.getContentPane().add(browser, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
this.getContentPane().add(buttonPanel, BorderLayout.NORTH);
final JTextField textField = new JTextField(30);
String userDir = System.getProperty("user.dir");
textField.setText("http://www.intrinsyc.com");
final JLabel label = new JLabel("Address");
JButton button = new JButton("Go");
buttonPanel.add(label);
buttonPanel.add(textField);
buttonPanel.add(button);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent event) {
System.out.println("url = " + textField.getText());
try {
browser.navigate(textField.getText(), null, null, null, null);
} catch(Exception e) {
e.printStackTrace();
}
}
});
setVisible(true);
} catch(Throwable e) {
e.printStackTrace();
}
}
class WebBrowserListener extends DWebBrowserEvents2Adapter {
public void beforeNavigate2(DWebBrowserEvents2BeforeNavigate2Event theEvent)
throws java.io.IOException, com.linar.jintegra.AutomationException {
}
public void navigateComplete2(DWebBrowserEvents2NavigateComplete2Event theEvent)
throws java.io.IOException, com.linar.jintegra.AutomationException {
System.out.println("Navigation complete " + theEvent.getURL());
}
public void onQuit(DWebBrowserEvents2OnQuitEvent theEvent) {
synchronized (this) {
com.linar.jintegra.Cleaner.releaseAll();
notify();
}
}
}
public static void main(String[] args) {
com.linar.jintegra.Log.logImmediately(3, "jintegra.log");
com.linar.jintegra.AuthInfo.setDefault("ITC", "rhe", "raymond");
WebBrowserExample browser = new WebBrowserExample();
}
} |
Remember to include the \jre\bin directory in your PATH environment variable:
set PATH=C:\Program Files\Java\j2sdk1.4.2\bin;C:\Program Files\Java\j2sdk1.4.2\jre\bin;C:\Program Files\J-Integra\COM\bin;%PATH%
To open an Office document, run the above code, and type the file name (with path) in the Address field, and then click the Go button to open the file (just like you would do in Internet Explorer).
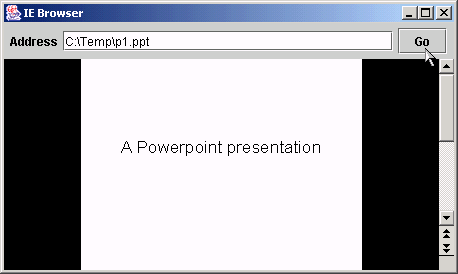
Note: If Office 2007 is installed, the Office document will not open inside the WebBrowser frame and a separate application window (Word, Excel, Powerpoint, etc.) will be launched instead. According to Microsoft, this is by design, but a workaround is provided in their Knowledge Base article 927009.
2. Use the Microsoft Office Web Component Object ActiveX Control
The Spreadsheet object contained in the Microsoft Office Web Components (OWC) Object is similar to the Excel WorkSheet. See the related article below for an example of embedding OWC in a Java frame.
3. Use a third party's ActiveX Control
There may be some third party's ActiveX Controls available for viewing Microsoft Office documents. Or you can build your own ActiveX Control for hosing Office documents, and there are two Microsoft Knowledge Base articles provide such an example:
For instance, if all the methods of Microsoft Word ActiveX document are wrapped and exposed in your custom built ActiveX Control, you can then embed your own ActiveX Control in a Java frame, and the Java code will be able to access those wrapper methods that talk to the original methods of the Word ActiveX document.
Related Article
#30421 : Embedding Excel as an ActiveX Control in a Java Frame