Class com.linar.ocxhost.Container
java.lang.Object
|
+----com.linar.ocxhost.Container
- public class Container extends Object
A thin ActiveX Control container, designed to render ActiveX Controls
accessible via DCOM.
Acrobat[tm]
example
// Assumes you have Acrobat installed, and that you have run 'com2java' on
// "\Acrobat3\Reader\ActiveX\pdf.tlb" specifying the package to be 'pdf'.
// Run ocxhost.exe once to install it, prior to running this example.
public class OcxHostTest {
public static void main(String[] args) throws Exception {
try {
com.linar.ocxhost.Container ocxHost = new com.linar.ocxhost.Container();
ocxHost.setVisible(true);
pdf.Pdf pdf = new pdf.Pdf(ocxHost.create("PDF.PdfCtrl.1"));
pdf.setSrc("C:\\mydir\\myfile.pdf");
Thread.sleep(10000);
} finally {
com.linar.jintegra.Cleaner.releaseAll();
}
}
} |
RealAudio[tm]
example
// Assumes you have RealAudio G2 installed, and that you have run 'com2java'
// on "rmoc3260.dll" specifying the package to be 'real'.
// Run ocxhost.exe once to install it, prior to running this example.
public class RealAudioTest {
public static void main(String[] args) throws Exception {
try {
com.linar.ocxhost.Container ocxHost = new com.linar.ocxhost.Container();
real.RealAudio real = new real.RealAudio(ocxHost.create("rmocx.RealPlayer G2 Control"));
ocxHost.move(100, 100, 300, 300);
ocxHost.setVisible(true);
real.setSource("http://j-integra.intrinsyc.com/");
real.doPlay();
Thread.sleep(15000);
} finally {
com.linar.jintegra.Cleaner.releaseAll();
}
}
} |

-
Container()
-
Constructs an ocxhost.Container on the local host
-
Container(String)
-
Construct a ocxhost.Container on specified host

-
create(String)
-
create an ActiveX Control and host it in this container.
-
move(int, int, int, int)
-
move/resize the container.
-
setVisible(boolean)
-
Make the container visible or invisible.

Container
public Container() throws IOException, UnknownHostException
-
Constructs a ocxhost.Container on the local host.
-
- Throws:
IOException
-
if there are problems communicating via DCOM
- Throws:
UnknownHostException
-
if the host can not be found
Container
public Container(String host) throws IOException, UnknownHostException
-
Construct a ocxhost.Container on specified host
-
- Parameters:
-
host - the host on which the object should be created
- Throws:
IOException
-
if there are problems communicating via DCOM
- Throws:
UnknownHostException
-
if the host can not be found
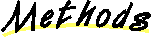
create
public Object create(String progId) throws IOException, AutomationException
-
create. Creates an ActiveX Control in the container. Pass a COM ProgId
(such as "PDF.PdfCtrl") or a COM class ID as a parameter.
-
- Parameters:
-
progId - The progId or class Id of the ActiveX Control to be created.
- Returns:
-
return value. A reference to the ActiveX Control that was created.
Wrap it in the appropriate generated proxy
- Throws:
IOException
-
If there are communications problems.
- Throws:
AutomationException
-
If the remote server throws an exception.
setVisible
public void setVisible(boolean v) throws IOException, AutomationException
-
setVisible. Make the container visible or invisible (initially it is
invisible).
-
- Parameters:
-
v - Whether the container should be visible.
- Throws:
IOException
-
If there are communications problems.
- Throws:
AutomationException
-
If the remote server throws an exception.
move
public void move(int x,
int y,
int width,
int height) throws IOException, AutomationException
-
move. Move the container to a specific location, and resize it.
-
- Parameters:
-
x - The x position
-
y - The y position
-
width - The width
-
height - The height
- Throws:
IOException
-
If there are communications problems.
- Throws:
AutomationException
-
If the remote server throws an exception.